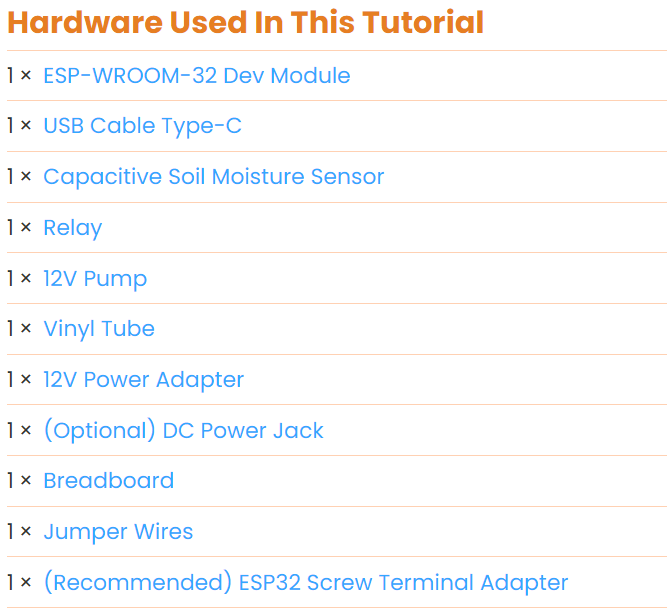
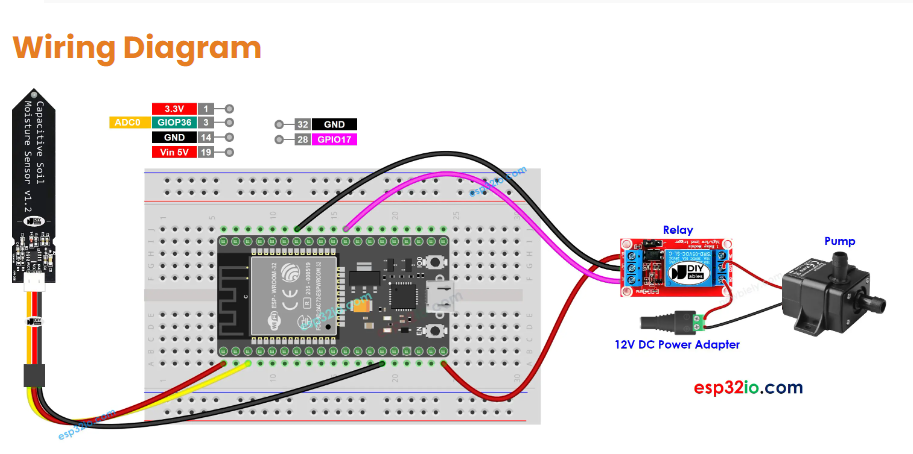
/*
* This ESP32 code is created by esp32io.com
*
* This ESP32 code is released in the public domain
*
* For more detail (instruction and wiring diagram), visit https://esp32io.com/tutorials/esp32-soil-moisture-sensor-pump
*/
#define RELAY_PIN 17 // ESP32 pin GPIO17 that connects to relay
#define MOISTURE_PIN 36 // ESP32 pin GPIO36 (ADC0) that connects to AOUT pin of moisture sensor
#define THRESHOLD 500 // => CHANGE YOUR THRESHOLD HERE
void setup() {
Serial.begin(9600);
pinMode(RELAY_PIN, OUTPUT);
}
void loop() {
int value = analogRead(MOISTURE_PIN); // read the analog value from soild moisture sensor
if (value < THRESHOLD) {
Serial.print("The soil moisture is DRY => activate pump");
digitalWrite(RELAY_PIN, HIGH);
} else {
Serial.print("The soil moisture is WET=> deactivate the pump");
digitalWrite(RELAY_PIN, LOW);
}
Serial.print(" (");
Serial.print(value);
Serial.println(")");
delay(1000);
}
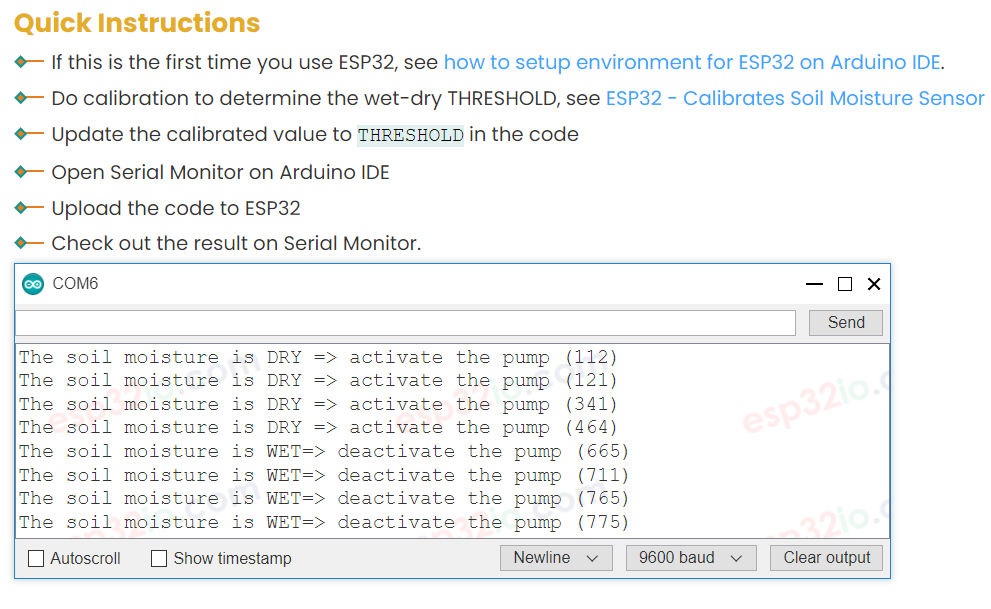
https://esp32io.com/tutorials/esp32-soil-moisture-sensor-pum
const int potPin = 2;
// variable for storing the potentiometer value
int potValue = 0;
void setup() {
analogReadResolution(8);
Serial.begin(115200);
delay(1000);
}
void loop() {
// Reading potentiometer value
potValue = analogRead(potPin);
Serial.println(potValue);
delay(500);
}