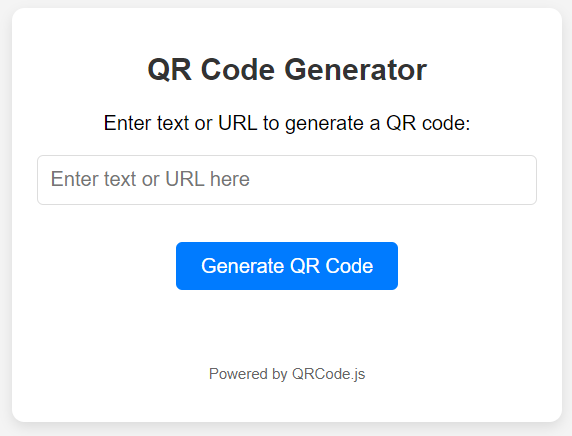
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Generate QR Code</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding-top: 50px;
}
#qrcode {
margin-top: 20px;
}
</style>
</head>
<body>
<h1>QR Code Generator</h1>
<p>Enter text to generate QR code:</p>
<input type="text" id="text-input" placeholder="Enter text or URL">
<button onclick="generateQRCode()">Generate QR Code</button>
<div id="qrcode"></div>
<!-- Include the QRCode.js library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/qrcodejs/1.0.0/qrcode.min.js"></script>
<script>
function generateQRCode() {
// Clear previous QR code
document.getElementById('qrcode').innerHTML = "";
// Get input text
var text = document.getElementById("text-input").value;
// Generate QR code
if (text) {
var qrcode = new QRCode(document.getElementById("qrcode"), {
text: text,
width: 128, // QR code width
height: 128, // QR code height
});
} else {
alert("Please enter text or URL");
}
}
</script>
</body>
</html>
วิธีการทำงานของโค้ด:
- QRCode.js เป็นไลบรารี JavaScript ที่ใช้สร้าง QR code บนหน้าเว็บ โดยต้องใส่
<script>
เพื่อดึงไลบรารีมาใช้ในไฟล์ HTML ของคุณจาก CDN (ที่เก็บไฟล์ออนไลน์). - เมื่อผู้ใช้กรอกข้อความหรือ URL แล้วกดปุ่ม “Generate QR Code” ระบบจะสร้าง QR code โดยแสดงผลใน
<div>
ที่มี idqrcode
.
การใช้งาน:
- เปิดไฟล์ HTML นี้ในเว็บเบราว์เซอร์ ใส่ข้อความหรือ URL ที่ต้องการ แล้วกดปุ่ม “Generate QR Code” เพื่อสร้าง QR code ได้ทันที.
หากต้องการปรับแต่งขนาดหรือสีของ QR code สามารถปรับค่าใน QRCode()
ได้ตามความต้องการครับ!
ตัวอย่าง Source Code HTML สำหรับการ generate QR code พร้อมการออกแบบ CSS ที่สวยงามและมี ปุ่มสำหรับบันทึก QR code ลงในเครื่อง:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>QR Code Generator</title>
<style>
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
background-color: #fff;
padding: 20px;
box-shadow: 0 4px 10px rgba(0, 0, 0, 0.1);
border-radius: 10px;
text-align: center;
max-width: 400px;
width: 100%;
}
h1 {
font-size: 24px;
color: #333;
margin-bottom: 20px;
}
input[type="text"] {
width: 100%;
padding: 10px;
font-size: 16px;
border: 1px solid #ddd;
border-radius: 5px;
margin-bottom: 20px;
box-sizing: border-box;
}
button {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 16px;
margin: 10px;
}
button:hover {
background-color: #0056b3;
}
#qrcode {
margin-top: 20px;
display: flex;
justify-content: center;
}
#save-btn {
display: none;
}
footer {
margin-top: 30px;
font-size: 12px;
color: #666;
}
</style>
</head>
<body>
<div class="container">
<h1>QR Code Generator</h1>
<p>Enter text or URL to generate a QR code:</p>
<input type="text" id="text-input" placeholder="Enter text or URL here">
<button onclick="generateQRCode()">Generate QR Code</button>
<button id="save-btn" onclick="saveQRCode()">Save QR Code</button>
<div id="qrcode"></div>
<footer>
<p>Powered by QRCode.js</p>
</footer>
</div>
<!-- Include the QRCode.js library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/qrcodejs/1.0.0/qrcode.min.js"></script>
<script>
function generateQRCode() {
// Clear previous QR code
document.getElementById('qrcode').innerHTML = "";
// Get input text
var text = document.getElementById("text-input").value;
// Generate QR code
if (text) {
var qrcode = new QRCode(document.getElementById("qrcode"), {
text: text,
width: 150, // QR code width
height: 150, // QR code height
colorDark : "#000000",
colorLight : "#ffffff",
correctLevel : QRCode.CorrectLevel.H
});
// Show save button after generating QR code
document.getElementById('save-btn').style.display = "inline-block";
} else {
alert("Please enter text or URL");
}
}
function saveQRCode() {
// Get the QR code canvas
var qrCanvas = document.querySelector('#qrcode canvas');
if (qrCanvas) {
// Create a link element
var link = document.createElement('a');
link.href = qrCanvas.toDataURL("image/png"); // Convert canvas to image data
link.download = 'qrcode.png'; // Set download file name
// Trigger the download
link.click();
} else {
alert("No QR code to save!");
}
}
</script>
</body>
</html>